In this tutorial, we will write a Java program to rotate a matrix by 90 degrees in clockwise rotation.
The clockwise rotation is also called as the right rotation of the matrix.
Rotate Matrix by 90 Degree Clockwise Algorithm:-
Step 1: Enter the number of rows (r).
Step 2: Enter the number of columns (c).
Step 3: Enter the elements of Matrix (arr[i][j]).
Step 4: Swap the elements of the first column with the last column (if matrix is of 3*3 order). The second column remains the same.
Step 5: Print the "Rotated Matrix".
Note: Rotation is possible if and only if matrix have the same number of rows and columns.
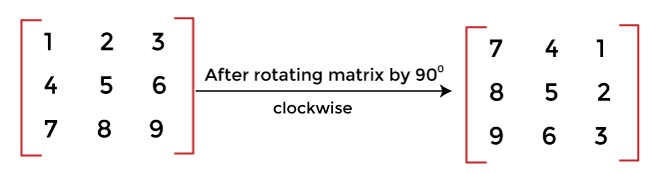
Now, Let's understand through an example. Suppose, the given matrix is :
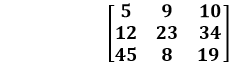
Let's find the transpose of the above matrix.
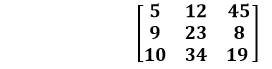
To rotate matrix, swap the first column of matrix with the last column of matrix.
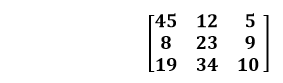
The above matrix is now a rotated matrix by 90 degrees.
If the given matrix is 4*4 matrix, swap the first column with the last column and the second column with the third column. For example, consider the following figure.
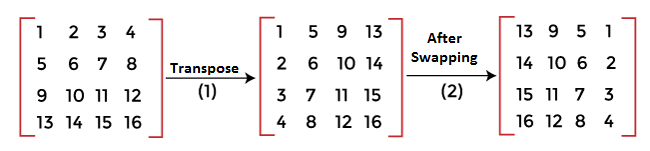
Rotate Matrix 90 Degrees Clockwise
import java.util.*;
class Clockwise_Rotate
{
public static void main()
{
Scanner sc=new Scanner(System.in);
System.out.println("Enter the number of Rows");
int r=sc.nextInt();
System.out.println("Enter the number of Columns");
int c=sc.nextInt();
int arr[][]=new int[r][c];
System.out.println("Enter the elements of Matrix");
for(int i=0;i<r;i++)
{
for(int j=0;j<c;j++)
{
arr[i][j]=sc.nextInt();
}
}
System.out.println("Input Matrix Is:");
for(int i=0;i<r;i++)
{
for(int j=0;j<c;j++)
{
System.out.print(arr[i][j]+" ");
}
System.out.println("");
}
System.out.println("Matrix After Rotation Is:");
for(int j=0;j<c;j++)
{
for(int i=r-1;i>=0;i--)
{
System.out.print(arr[i][j]+"\t");
}
System.out.println(" ");
}
}
}
-------------
OUTPUT:-
-------------
Enter the number of Rows
4
Enter the number of Columns
4
Enter the elements of Matrix
1
5
6
4
2
3
5
7
8
9
3
1
6
4
5
2
Input Matrix Is:
1 5 6 4
2 3 5 7
8 9 3 1
6 4 5 2
Matrix After Rotation is:
6 8 2 1
4 9 3 5
5 3 5 6
2 1 7 4
1 Comments
Fabulous... You are doing well 😃
ReplyDeleteJust write down your question to get the answer...